#!/usr/bin/env bash
# it searches in the current working directory
function calculate_size
# takes a path as input
{
du -mc "$1" | tail -1 | awk '{print $1}'
}
function list_dirs
{
# find . -depth 1 -type d | while read -r line; do
find . -maxdepth 1 -type d | while read -r line; do
printf "$my_format" "$(calculate_size "$line")" "mb" "$line"
done
}
function make_divider
{
nr=$(tput cols)
for i in `seq 1 $nr`; do printf "-";done
}
function my_main
{
my_format="%9s\t%2s\t %s\n"
nr=$(tput cols)
my_divider=$(make_divider)
clear
echo
echo "$my_divider"
echo
echo "Dirs: "$(pwd)
printf "\n"
echo "$my_divider"
list_dirs | sort -r -nk1 2>/dev/null
echo "$my_divider"
printf "\n\n"
}
# ===========================================================
# Now follows the actual program
my_main
I made this to list directory size in bash in a custom way
What does the the script do:
- It calculates the size of all subdirectories
- Sorts the outcome on size
- Makes a list
How to use it:
- Make the script executable with chmod +x
- In terminal. change to the directory you want to examine
- Copy the script into this directory and run it.Â
- Or past the lines of script and press enter
- Or call the script from your directoryÂ
HP
Example of the script output:
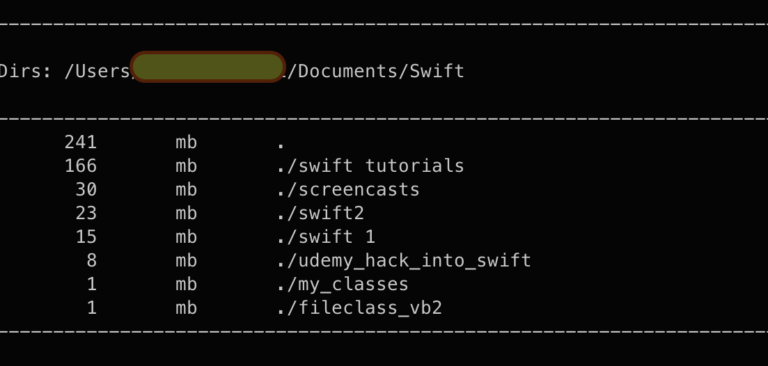